Python is an indispensable tool for data science professionals, playing a pivotal role in data analysis, machine learning, and scientific computing. Whether you’re a novice or an experienced practitioner, enhancing your Python programming skills is an ongoing journey. This article is your gateway to 14 exciting Python project ideas, each carefully crafted to cater to the needs of data science enthusiasts. These projects offer a unique opportunity to not only improve your Python skills but also create practical applications that can be applied in your data-driven endeavors.
So, let’s begin our Python project journey!
Top 14 Mini Python Projects
Calculator
A beginner-friendly Python project idea is to create a basic calculator. This program performs fundamental mathematical operations, such as addition, subtraction, multiplication, and division. You can further enhance it by adding features like memory functions or history tracking. Building a calculator is a great way to practice Python’s core syntax and mathematical operations.
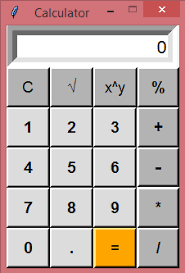
Python Code
def add(x, y): return x + y def subtract(x, y): return x - y def multiply(x, y): return x * y def divide(x, y): if y == 0: return "Cannot divide by zero" return x / y while True: print("Options:") print("Enter 'add' for addition") print("Enter 'subtract' for subtraction") print("Enter 'multiply' for multiplication") print("Enter 'divide' for division") print("Enter 'exit' to end the program") user_input = input(": ") if user_input == "exit": break if user_input in ("add", "subtract", "multiply", "divide"): num1 = float(input("Enter first number: ")) num2 = float(input("Enter second number: ")) if user_input == "add": print("Result: ", add(num1, num2)) elif user_input == "subtract": print("Result: ", subtract(num1, num2)) elif user_input == "multiply": print("Result: ", multiply(num1, num2)) elif user_input == "divide": print("Result: ", divide(num1, num2)) else: print("Invalid input")
To-Do List
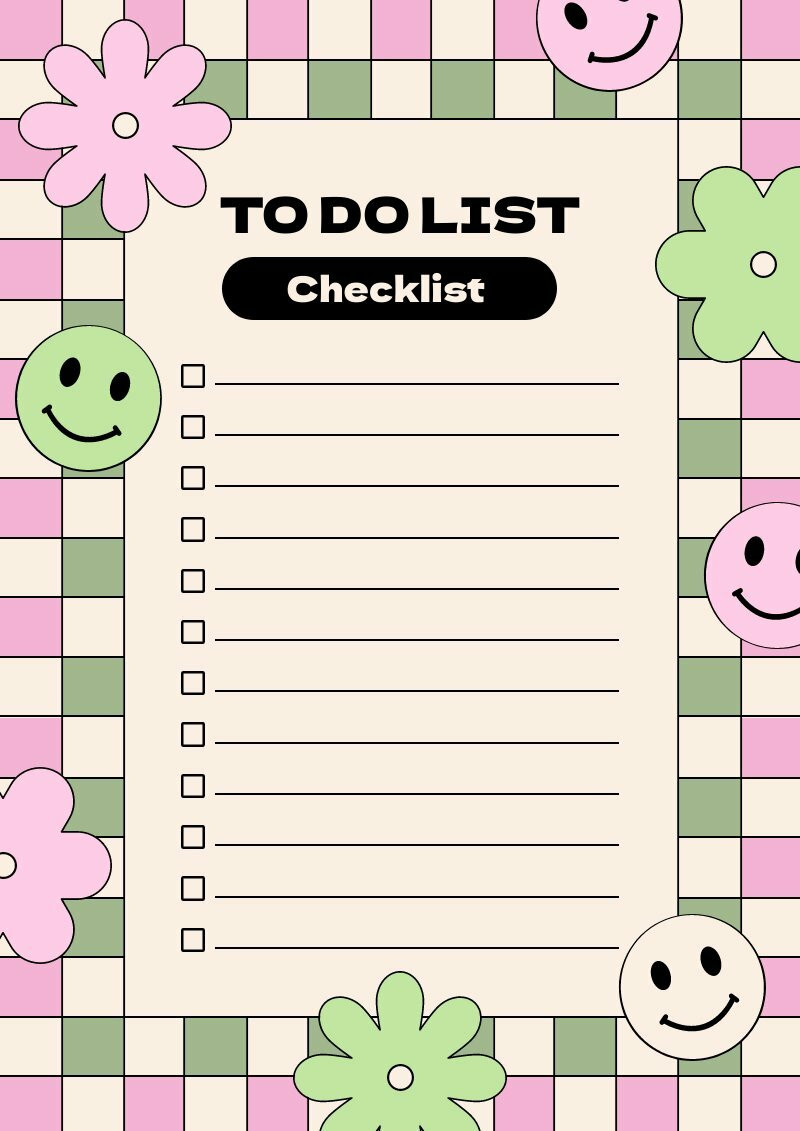
A to-do list application is a handy tool for organizing tasks. Create a basic Python program that allows users to add, delete, and view tasks. This simple project helps beginners understand data storage and manipulation. As you progress, you can enhance it with features like due dates, priorities, and more, making it a valuable tool for personal task management.
Python Code
# Define an empty list to store tasks
tasks = [] def add_task(task): tasks.append(task) print("Task added:", task) def delete_task(task): if task in tasks: tasks.remove(task) print("Task deleted:", task) else: print("Task not found") def view_tasks(): if not tasks: print("No tasks in the list") else: print("Tasks:") for index, task in enumerate(tasks, start=1): print(f"{index}. {task}") while True: print("Options:") print("Enter 'add' to add a task") print("Enter 'delete' to delete a task") print("Enter 'view' to view tasks") print("Enter 'exit' to end the program") user_input = input(": ") if user_input == "exit": break elif user_input == "add": task = input("Enter a task: ") add_task(task) elif user_input == "delete": task = input("Enter the task to delete: ") delete_task(task) elif user_input == "view": view_tasks() else: print("Invalid input")
Guess the Number
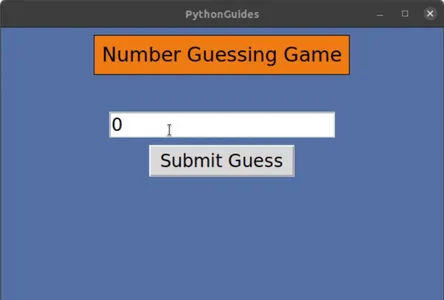
“Guess the Number” is a classic Python project where the computer selects a random number, and the player’s task is to guess it. Players can input their guesses, and the program provides hints, progressively challenging the game. It’s an engaging and educational project that enhances your understanding of random number generation and user interactions.
Python Code
import random # Generate a random number between 1 and 100
secret_number = random.randint(1, 100)
attempts = 0 print("Welcome to the Guess the Number game!")
print("I'm thinking of a number between 1 and 100.") while True: try: guess = int(input("Your guess: ")) attempts += 1 if guess < secret_number: print("Try a higher number.") elif guess > secret_number: print("Try a lower number.") else: print(f"Congratulations! You've guessed the number in {attempts} attempts.") break except ValueError: print("Invalid input. Please enter a number between 1 and 100.") print("Thank you for playing!")
Basic Web Scraper
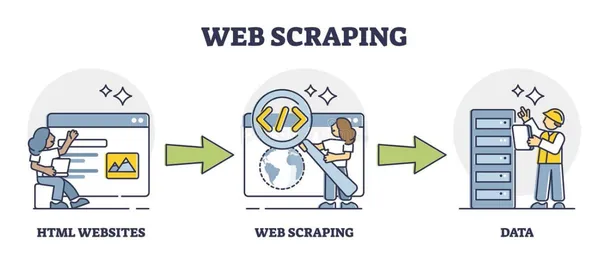
Create a basic web scraper in Python to extract data from websites. This project helps you understand web scraping concepts and HTTP requests. Start by fetching information from a simple webpage and gradually progress to more complex scraping tasks. This project offers valuable insights into data acquisition and manipulation using Python.
Python Code
import requests
from bs4 import BeautifulSoup # URL of the webpage you want to scrape
url = "https://example.com" # Send an HTTP GET request to the URL
response = requests.get(url) # Check if the request was successful (status code 200)
if response.status_code == 200: # Parse the HTML content of the page using BeautifulSoup soup = BeautifulSoup(response.text, 'html.parser') # Extract data from the webpage (for example, scraping all the links) links = [] for link in soup.find_all('a'): links.append(link.get('href')) # Print the scraped data (in this case, the links) for link in links: print(link)
else: print("Failed to fetch the webpage. Status code:", response.status_code)
Word Counter
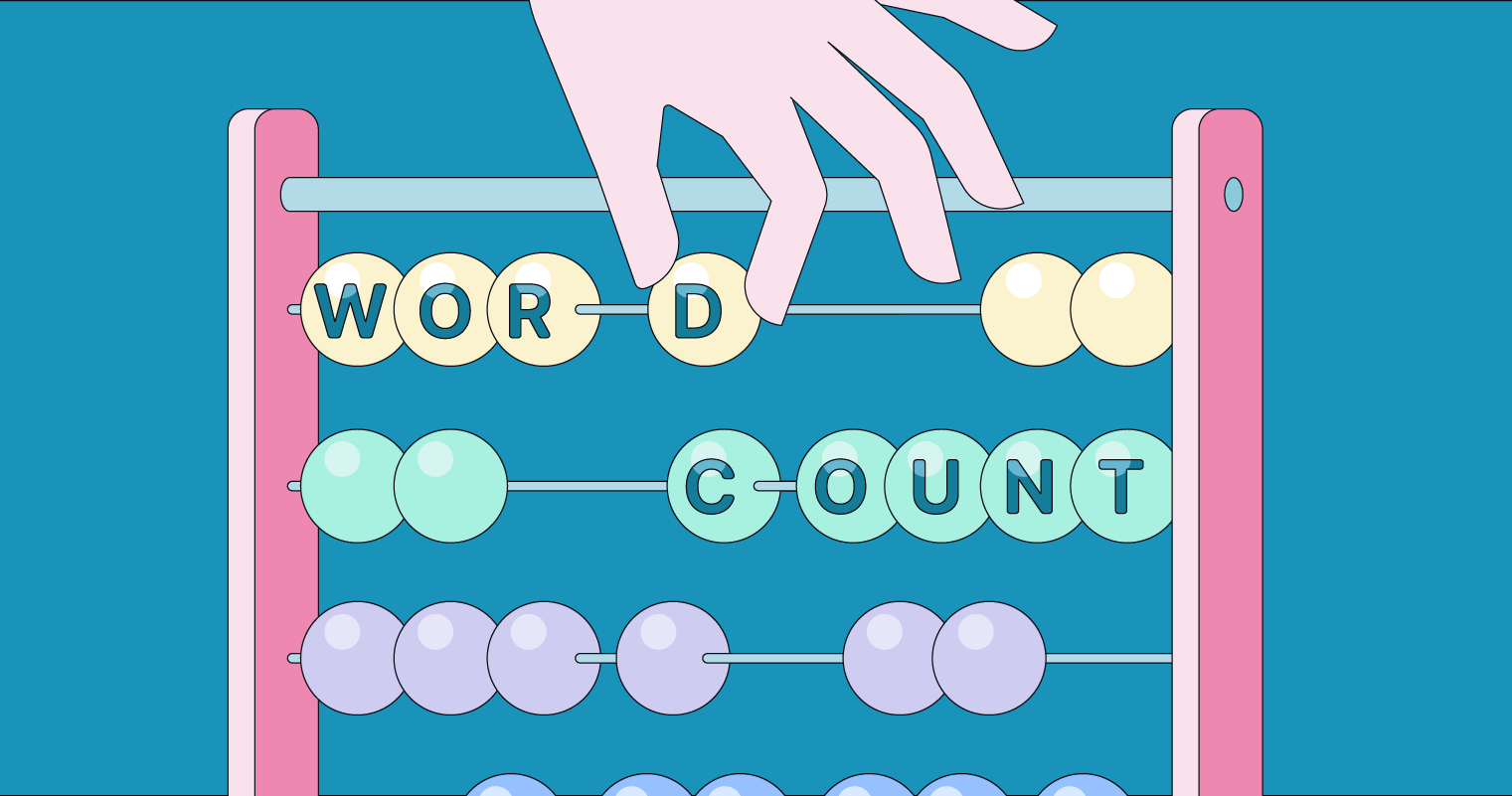
A Word Counter is a simple Python project where you create a program to count the number of words in a text. It’s an excellent exercise for beginners, helping them learn about string manipulation and basic text analysis. You can later expand it to count characters, sentences, or paragraphs for a more versatile tool.
Python Code
def count_words(text): # Split the text into words using whitespace as a delimiter words = text.split() return len(words) # Get input text from the user
text = input("Enter some text: ") # Call the count_words function to count the words
word_count = count_words(text) # Display the word count
print(f"Word count: {word_count}")
Hangman Game
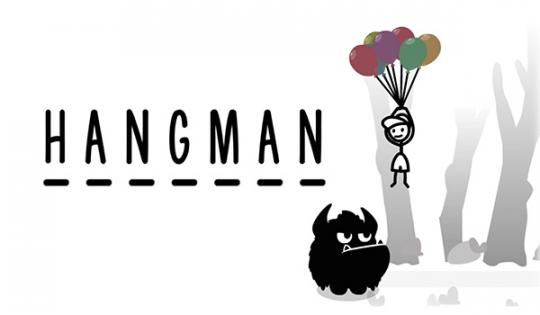
The Hangman Game is a classic word-guessing pastime brought to life in Python. In this engaging project, players attempt to guess a word letter by letter. The program can include diverse word selections and even a scoring system, making it an entertaining and educational project for beginners.
Python Code
import random # List of words to choose from
words = ["python", "hangman", "computer", "programming", "challenge"] # Function to choose a random word
def choose_word(): return random.choice(words) # Function to display the current state of the word with blanks
def display_word(word, guessed_letters): display = "" for letter in word: if letter in guessed_letters: display += letter else: display += "_" return display # Function to play Hangman
def play_hangman(): word_to_guess = choose_word() guessed_letters = [] attempts = 6 print("Welcome to Hangman!") while attempts > 0: print("nWord: " + display_word(word_to_guess, guessed_letters)) print("Attempts left:", attempts) guess = input("Guess a letter: ").lower() if len(guess) == 1 and guess.isalpha(): if guess in guessed_letters: print("You've already guessed that letter.") elif guess in word_to_guess: print("Good guess!") guessed_letters.append(guess) if set(word_to_guess).issubset(set(guessed_letters)): print("Congratulations! You've guessed the word:", word_to_guess) break else: print("Wrong guess!") guessed_letters.append(guess) attempts -= 1 else: print("Invalid input. Please enter a single letter.") if attempts == 0: print("You ran out of attempts. The word was:", word_to_guess) # Start the game
play_hangman()
Simple Alarm Clock
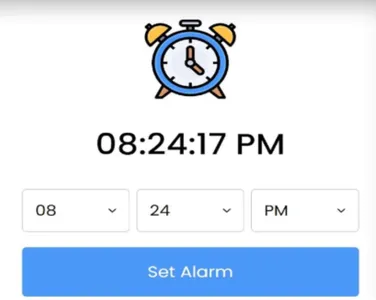
A Simple Alarm Clock project involves creating a Python application that allows users to set alarms, with features like snooze and stop options. It’s an excellent project for beginners to delve into time and date handling in Python. This hands-on experience can lay the foundation for more complex applications and help users gain practical programming skills.
Python Code
import time
import winsound # Function to set an alarm
def set_alarm(): alarm_time = input("Enter the alarm time (HH:MM): ") while True: current_time = time.strftime("%H:%M") if current_time == alarm_time: print("Wake up!") winsound.Beep(1000, 1000) # Beep for 1 second break # Function to snooze the alarm
def snooze_alarm(): snooze_time = 5 # Snooze for 5 minutes alarm_time = time.time() + snooze_time * 60 while time.time() < alarm_time: pass print("Snooze time is over. Wake up!") winsound.Beep(1000, 1000) # Beep for 1 second # Function to stop the alarm
def stop_alarm(): print("Alarm stopped") # Main loop for the alarm clock
while True: print("Options:") print("Enter '1' to set an alarm") print("Enter '2' to snooze the alarm") print("Enter '3' to stop the alarm") print("Enter '4' to exit") choice = input(": ") if choice == '1': set_alarm() elif choice == '2': snooze_alarm() elif choice == '3': stop_alarm() elif choice == '4': break else: print("Invalid input. Please enter a valid option.")
Dice Roller

The Dice Roller project is a fun Python endeavor that simulates rolling dice. Random number generation allows users to roll various types of dice, from standard six-sided ones to more exotic varieties. It’s a simple yet entertaining way to delve into Python’s randomization capabilities and create an interactive dice-rolling experience.
Python Code
import random def roll_dice(sides): return random.randint(1, sides) while True: print("Options:") print("Enter 'roll' to roll a dice") print("Enter 'exit' to end the program") user_input = input(": ") if user_input == "exit": break if user_input == "roll": sides = int(input("Enter the number of sides on the dice: ")) result = roll_dice(sides) print(f"You rolled a {sides}-sided dice and got: {result}") else: print("Invalid input. Please enter a valid option.")
Mad Libs Generator
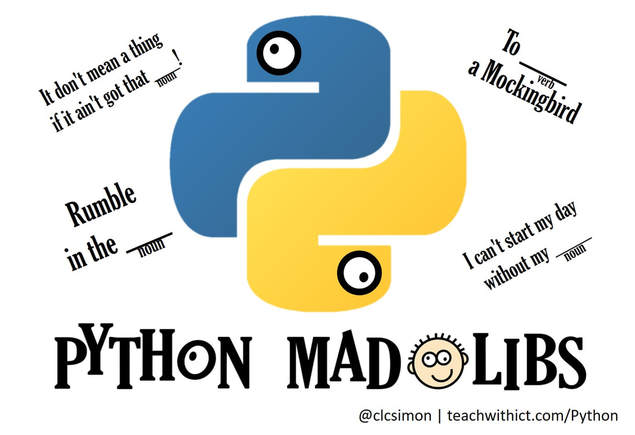
The Mad Libs Generator is a creative and entertaining Python project. It prompts users to input various word types (nouns, verbs, adjectives) and then generates amusing stories using their words. This project is fun and an excellent exercise in string manipulation and user interaction. It’s a playful way to explore the world of Python programming.
Python Code
# Define a Mad Libs story template
mad_libs_template = "Once upon a time, in a {adjective} {place}, there lived a {animal}. It was a {adjective} and {color} {animal}. One day, the {animal} {verb} to the {place} and met a {adjective} {person}. They became fast friends and went on an adventure to {verb} the {noun}." # Create a dictionary to store user inputs
user_inputs = {} # Function to get user inputs
def get_user_input(prompt): value = input(prompt + ": ") return value # Replace placeholders in the story template with user inputs
def generate_mad_libs_story(template, inputs): story = template.format(**inputs) return story # Get user inputs for the Mad Libs
for placeholder in ["adjective", "place", "animal", "color", "verb", "person", "noun"]: user_inputs[placeholder] = get_user_input(f"Enter a {placeholder}") # Generate the Mad Libs story
mad_libs_story = generate_mad_libs_story(mad_libs_template, user_inputs) # Display the generated story
print("nHere's your Mad Libs story:")
print(mad_libs_story)
Password Generator
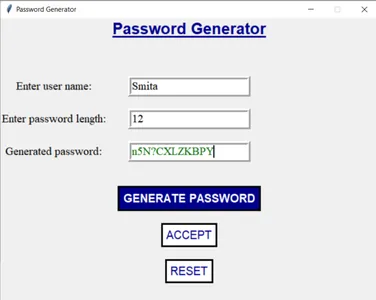
The Password Generator project involves creating a Python program that generates strong, secure passwords based on user preferences. Users can specify parameters such as password length and character types, and the program will output a robust password, enhancing cybersecurity for online accounts and personal data.
Python Code
import random
import string def generate_password(length, use_uppercase, use_digits, use_special_chars): characters = string.ascii_lowercase if use_uppercase: characters += string.ascii_uppercase if use_digits: characters += string.digits if use_special_chars: characters += string.punctuation if len(characters) == 0: print("Error: No character types selected. Please enable at least one option.") return None password = ''.join(random.choice(characters) for _ in range(length)) return password def main(): print("Password Generator") while True: length = int(input("Enter the password length: ")) use_uppercase = input("Include uppercase letters? (yes/no): ").lower() == "yes" use_digits = input("Include digits? (yes/no): ").lower() == "yes" use_special_chars = input("Include special characters? (yes/no): ").lower() == "yes" password = generate_password(length, use_uppercase, use_digits, use_special_chars) if password: print("Generated Password:", password) another = input("Generate another password? (yes/no): ").lower() if another != "yes": break if __name__ == "__main__": main()
Basic Text Editor
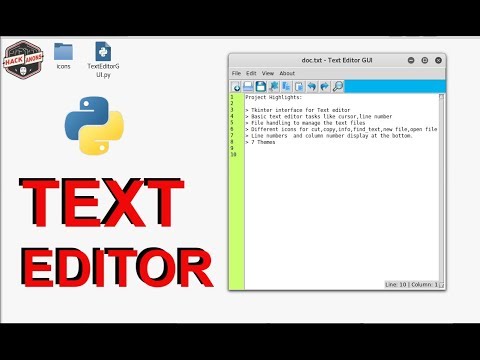
A basic text editor is a simple software application for creating and editing plain text documents. It offers essential functions like typing, copying, cutting, pasting, and basic formatting. While lacking advanced features in word processors, it is lightweight, quick to use, and suitable for tasks like note-taking or writing code. Popular examples include Notepad (Windows) and TextEdit (macOS), providing a user-friendly environment for minimalistic text manipulation.
Python Code
import tkinter as tk
from tkinter import filedialog def new_file(): text.delete(1.0, tk.END) def open_file(): file_path = filedialog.askopenfilename(filetypes=[("Text Files", "*.txt")]) if file_path: with open(file_path, "r") as file: text.delete(1.0, tk.END) text.insert(tk.END, file.read()) def save_file(): file_path = filedialog.asksaveasfilename(defaultextension=".txt", filetypes=[("Text Files", "*.txt")]) if file_path: with open(file_path, "w") as file: file.write(text.get(1.0, tk.END)) # Create the main window
root = tk.Tk()
root.title("Basic Text Editor") # Create a menu
menu = tk.Menu(root)
root.config(menu=menu) file_menu = tk.Menu(menu)
menu.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="New", command=new_file)
file_menu.add_command(label="Open", command=open_file)
file_menu.add_command(label="Save", command=save_file)
file_menu.add_separator()
file_menu.add_command(label="Exit", command=root.quit) # Create a text area
text = tk.Text(root, wrap=tk.WORD)
text.pack(expand=True, fill="both") # Start the GUI main loop
root.mainloop()
Mini Weather App
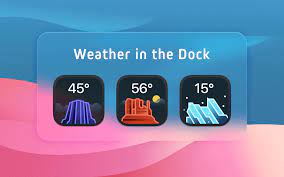
The Mini Weather App is a compact tool for quick weather updates. Users can access current conditions and forecasts in a concise interface. It provides essential information like temperature, precipitation, and wind speed, ensuring users stay informed on the go. This lightweight app is perfect for those seeking immediate weather insights without the clutter.
Python Code
import requests def get_weather(city, api_key): base_url = "https://api.openweathermap.org/data/2.5/weather" params = { "q": city, "appid": api_key, "units": "metric" # Change to "imperial" for Fahrenheit } response = requests.get(base_url, params=params) if response.status_code == 200: weather_data = response.json() temperature = weather_data["main"]["temp"] description = weather_data["weather"][0]["description"] humidity = weather_data["main"]["humidity"] wind_speed = weather_data["wind"]["speed"] print(f"Weather in {city}:") print(f"Temperature: {temperature}°C") print(f"Description: {description}") print(f"Humidity: {humidity}%") print(f"Wind Speed: {wind_speed} m/s") else: print("Failed to fetch weather data. Please check your city name and API key.") def main(): print("Mini Weather App") city = input("Enter a city: ") api_key = input("Enter your OpenWeatherMap API key: ") get_weather(city, api_key) if __name__ == "__main__": main()
Basic Paint Application
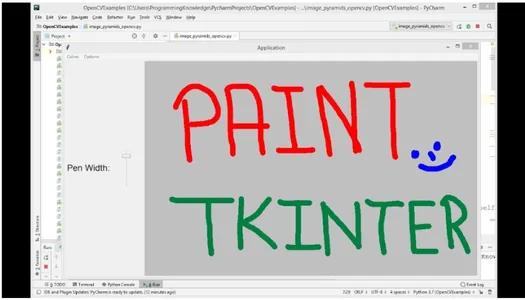
A basic paint application is a user-friendly software that allows users to create and edit digital images using various tools like brushes, colors, and shapes. It’s ideal for simple graphic design and digital art, offering essential drawing, coloring, and basic image manipulation features. While lacking advanced features, it is a great starting point for novice artists and designers.
Python Code
import tkinter as tk
from tkinter import colorchooser def start_paint(event): global prev_x, prev_y prev_x, prev_y = event.x, event.y def paint(event): x, y = event.x, event.y canvas.create_line((prev_x, prev_y, x, y), fill=current_color, width=brush_size, capstyle=tk.ROUND, smooth=tk.TRUE) prev_x, prev_y = x, y def choose_color(): global current_color color = colorchooser.askcolor()[1] if color: current_color = color def change_brush_size(new_size): global brush_size brush_size = new_size root = tk.Tk()
root.title("Basic Paint Application") current_color = "black"
brush_size = 2
prev_x, prev_y = None, None canvas = tk.Canvas(root, bg="white")
canvas.pack(fill=tk.BOTH, expand=True) canvas.bind("<Button-1>", start_paint)
canvas.bind("<B1-Motion>", paint) menu = tk.Menu(root)
root.config(menu=menu)
options_menu = tk.Menu(menu, tearoff=0)
menu.add_cascade(label="Options", menu=options_menu)
options_menu.add_command(label="Choose Color", command=choose_color)
options_menu.add_command(label="Brush Size (1)", command=lambda: change_brush_size(1))
options_menu.add_command(label="Brush Size (3)", command=lambda: change_brush_size(3))
options_menu.add_command(label="Brush Size (5)", command=lambda: change_brush_size(5)) root.mainloop()
Basic Chat Application
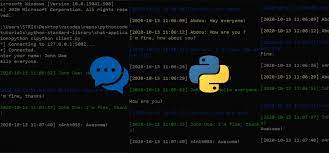
A basic chat application enables real-time text communication between users. Users can send and receive messages, creating one-on-one or group conversations. Features typically include message typing indicators, read receipts, and user profiles. These apps are popular for personal and business communication, fostering instant, convenient, and often asynchronous exchanges of information and ideas.
Server Python Code
import socket
import threading # Server configuration
HOST = '0.0.0.0' # Listen on all available network interfaces
PORT = 12345 # Create a socket for the server
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind((HOST, PORT))
server_socket.listen() clients = [] def handle_client(client_socket): while True: try: message = client_socket.recv(1024).decode("utf-8") if not message: remove_client(client_socket) else: broadcast(message, client_socket) except Exception as e: print(f"An error occurred: {str(e)}") remove_client(client_socket) break def remove_client(client_socket): if client_socket in clients: clients.remove(client_socket) def broadcast(message, client_socket): for client in clients: if client != client_socket: try: client.send(message.encode("utf-8")) except Exception as e: remove_client(client) print(f"An error occurred: {str(e)}") def main(): print("Chat server is listening on port", PORT) while True: client_socket, addr = server_socket.accept() clients.append(client_socket) client_handler = threading.Thread(target=handle_client, args=(client_socket,)) client_handler.start() if __name__ == "__main__": main()
Client Python Code
import socket
import threading # Client configuration
HOST = '127.0.0.1' # Server's IP address
PORT = 12345 # Create a socket for the client
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((HOST, PORT)) def receive_messages(): while True: try: message = client_socket.recv(1024).decode("utf-8") print(message) except Exception as e: print(f"An error occurred: {str(e)}") client_socket.close() break def send_messages(): while True: message = input() client_socket.send(message.encode("utf-8")) def main(): print("Connected to the chat server.") receive_thread = threading.Thread(target=receive_messages) receive_thread.start() send_thread = threading.Thread(target=send_messages) send_thread.start() if __name__ == "__main__": main()
Importance of Python in Data Science
Python holds a paramount position in the realm of data science due to its versatility, efficiency, and an extensive ecosystem of libraries and tools tailored for data analysis. Its importance lies in several key aspects:
- Ease of Learning and Use: Python’s simple and readable syntax makes it accessible for both beginners and experts, expediting the learning curve for data science practitioners.
- Abundant Libraries: Python boasts powerful libraries like NumPy for numerical operations, Pandas for data manipulation, Matplotlib and Seaborn for visualization, and Scikit-Learn for machine learning. This library-rich environment streamlines complex data analysis tasks.
- Community Support: The vast Python community continuously develops and maintains data science libraries. This ensures that tools are up-to-date, reliable, and backed by a supportive user community.
- Machine Learning and AI: Python serves as a hub for machine learning and AI development, offering libraries like TensorFlow, Keras, and PyTorch. These tools simplify the creation and deployment of predictive models.
- Data Visualization: Python enables the creation of compelling data visualizations, enhancing the understanding and communication of data insights.
- Integration: Python seamlessly integrates with databases, web frameworks, and big data technologies, making it an excellent choice for end-to-end data science pipelines.
Also Read: Top 10 Uses of Python in the Real World
Conclusion
These 14 small Python projects offer an excellent starting point for beginners to enhance their coding skills. They provide practical insights into Python’s core concepts. As you embark on your programming journey, remember that practice is crucial to mastery.
If you’re prepared to move forward, consider signing up for Analytics Vidhya’s Python course. You will gain in-depth education, practical experience, and the chance to work on actual data science projects. This course is even for beginners who have a coding or Data Science background. Take advantage of the opportunity to further your career and develop your Python skills. Join our Python course today!
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2023/10/small-python-projects/
- :is
- :not
- :where
- $UP
- 1
- 10
- 100
- 14
- 200
- 60
- a
- About
- access
- accessible
- Accounts
- acquisition
- actual
- add
- added
- adding
- addition
- address
- advanced
- ADvantage
- Adventure
- AI
- alarm
- All
- allows
- already
- also
- an
- analysis
- and
- animal
- Another
- api
- app
- Application
- applications
- applied
- apps
- ARE
- AREA
- Art
- article
- Artists
- AS
- aspects
- At
- attempt
- Attempts
- available
- backed
- background
- based
- basic
- BE
- became
- begin
- Beginners
- between
- Big
- Big Data
- Black
- boasts
- both
- Break
- brought
- Building
- business
- but
- by
- call
- CAN
- cannot
- canvas
- capabilities
- Career
- carefully
- case
- cater
- challenge
- challenging
- Chance
- change
- character
- characters
- check
- choice
- Choose
- City
- classic
- client
- clients
- Clock
- clutter
- code
- Code review
- Coding
- color
- COM
- Communication
- community
- compact
- compelling
- complex
- computer
- computing
- concepts
- concise
- conditions
- Configuration
- connected
- Consider
- content
- continuously
- Convenient
- conversations
- Cool
- copying
- Core
- Counter
- course
- crafted
- create
- Creating
- creation
- Creative
- crucial
- Current
- Current state
- curve
- cutting
- Cybersecurity
- data
- data analysis
- data science
- data storage
- data-driven
- databases
- Date
- Dates
- day
- define
- delve
- deployment
- description
- Design
- designers
- develop
- Development
- develops
- digital
- Digital Art
- digits
- Display
- diverse
- divide
- Division
- documents
- drawing
- due
- e
- each
- ecosystem
- editor
- Education
- educational
- efficiency
- else
- embark
- empty
- enable
- enables
- end
- end-to-end
- endeavor
- endeavors
- engaging
- enhance
- Enhances
- enhancing
- ensures
- ensuring
- Enter
- entertaining
- enthusiasts
- Environment
- error
- essential
- Ether (ETH)
- Even
- Event
- example
- examples
- excellent
- Except
- exception
- Exchanges
- exciting
- Exercise
- Exit
- Exotic
- Expand
- experience
- experienced
- experts
- explore
- extensive
- extract
- Failed
- FAST
- Features
- File
- Files
- First
- For
- forecasts
- Forward
- fostering
- found
- Foundation
- frameworks
- friends
- from
- fun
- function
- functions
- fundamental
- further
- Gain
- game
- gateway
- generate
- generated
- generates
- generation
- generator
- get
- Global
- Go
- good
- got
- gradually
- graphic
- great
- Group
- guessed
- Handling
- hands-on
- handy
- Have
- help
- helping
- helps
- hh
- High
- higher
- hints
- history
- holds
- host
- HTML
- http
- HTTPS
- Hub
- i
- idea
- ideal
- ideas
- if
- image
- images
- immediate
- Imperial
- import
- importance
- improve
- in
- in-depth
- include
- index
- Indicators
- information
- informed
- input
- inputs
- insights
- instant
- Integrates
- interaction
- interactions
- interactive
- Interface
- interfaces
- into
- involves
- IP
- IP Address
- IT
- ITS
- join
- journey
- keras
- Key
- later
- lay
- LEARN
- learning
- least
- left
- Length
- letter
- libraries
- lies
- Life
- lightweight
- like
- LINK
- links
- List
- Listening
- lower
- machine
- machine learning
- macos
- Main
- maintains
- MAKES
- Making
- management
- Manipulation
- math
- mathematical
- matplotlib
- max-width
- Memory
- Menu
- message
- messages
- met
- metric
- minimalistic
- minutes
- models
- more
- move
- move forward
- name
- needs
- network
- New
- no
- None
- Noun
- nouns
- novice
- number
- numerical
- numpy
- occurred
- of
- offer
- offering
- Offers
- often
- on
- once
- ONE
- ones
- ongoing
- online
- only
- open
- Operations
- Opportunity
- Option
- Options
- or
- organizing
- our
- out
- output
- over
- page
- paint
- pandas
- parameters
- Paramount
- pass
- Password
- Passwords
- perfect
- performs
- person
- personal
- personal data
- pivotal
- Place
- placeholder
- Plain
- plato
- Plato Data Intelligence
- PlatoData
- Play
- players
- playing
- please
- Point
- Popular
- position
- powerful
- Practical
- Practical Applications
- practice
- predictive
- preferences
- prepared
- processors
- professionals
- Profiles
- Program
- Programming
- Progress
- progressively
- project
- projects
- provide
- provides
- providing
- Python
- pytorch
- Quick
- R
- random
- Read
- real
- real world
- real-time
- realm
- receipts
- receive
- reliable
- remember
- replace
- request
- requests
- response
- result
- return
- review
- robust
- Role
- Roll
- Rolled
- Rolling
- root
- round
- s
- Save
- Science
- scientific
- scikit-learn
- scoring
- scraping
- seaborn
- seamlessly
- Second
- secure
- seeking
- selected
- send
- server
- serves
- set
- several
- shapes
- Sides
- signing
- Simple
- simplify
- simulates
- single
- Size
- skills
- small
- Software
- some
- special
- speed
- split
- stack
- standard
- start
- Starting
- State
- Status
- stay
- Stop
- stopped
- storage
- store
- Stories
- Story
- String
- strong
- successful
- such
- suitable
- support
- supportive
- syntax
- system
- tailored
- Take
- Task
- tasks
- Technologies
- template
- tensorflow
- text
- thank
- that
- The
- the world
- their
- Them
- then
- There.
- These
- they
- Thinking
- this
- those
- time
- to
- tool
- tools
- Topics
- Tracking
- true
- try
- types
- typically
- understand
- understanding
- unique
- units
- up-to-date
- Updates
- upon
- URL
- use
- User
- user-friendly
- users
- uses
- using
- valid
- Valuable
- value
- various
- Vast
- Ve
- versatile
- versatility
- View
- visualization
- W
- Wake
- want
- was
- Way..
- Weather
- web
- web scraping
- webp
- websites
- welcome
- went
- whether
- while
- white
- WHO
- will
- wind
- window
- windows
- with
- without
- Word
- words
- Work
- world
- writing
- Wrong
- X
- yes
- yet
- you
- Your
- youtube
- zephyrnet
- zero