Introduction
CTE is the short form for Common Table Expressions. CTE is one of the most powerful tools of SQL (Structured Query Language), and it also helps to clean the data. It is the concept of SQL (Structured Query Language) used to simplify coding and help to get the result as quickly as possible. CTE is the temporary table used to reference the original table. If the original table contains too many columns and we require only a few of them, we can make CTE (a temporary table) containing the required columns only.
CTE is used to solve complex problems easily with good readability of code. A code should always be easier to read so that if you are referring it after some time, you will get that code immediately. Even if the third person is referring to your code, he/she can understand it very well if the readability of your code is easy and good. CTE is one of the important concepts of SQL. If you have knowledge of CTE, you are stepping into the world of advanced SQL. Common Table Expressions (commonly known as CTE) helps Data analysts, data scientists, or any other analysts who want to extract their data efficiently and effectively from a table containing big data.
This article was published as a part of the Data Science Blogathon.
Table of Contents
Syntax of CTE
The following is the syntax of CTE:
WITH CTE_NAME AS
( SELECT column_name1, column_name2,..., column_nameN FROM table_name WHERE condition
)
SELECT column_name1, column_name2,..., column_nameN FROM CTE_NAME;
Example of CTE
Example 1:
Below code is executed in MySQL:
-- Creating databse
CREATE DATABASE employee_details; -- Use database
USE employee_details; -- Creating table
CREATE TABLE employee
(
emp_id INT PRIMARY KEY,
emp_name VARCHAR(20),
email_id VARCHAR(30),
city VARCHAR(25),
Verification_status CHAR(3)
); -- Inserting values into the table
INSERT INTO employee VALUES (1,'Suhas','[email protected]','Mumbai','Yes');
INSERT INTO employee VALUES (2,'Yohani','[email protected]','Mumbai','No');
INSERT INTO employee VALUES (3,'Reshama','[email protected]','Pune','Yes');
INSERT INTO employee VALUES (4,'Raj','[email protected]','Bangalore','No');
INSERT INTO employee VALUES (5,'Shivani','[email protected]','Bangalore','Yes');
SELECT * FROM employee;
The Output is given below:
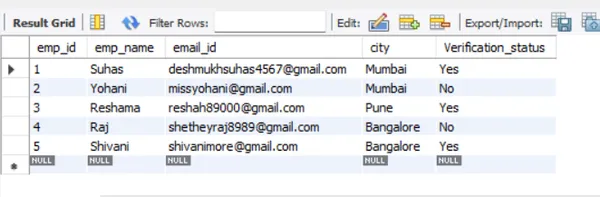
In the example above, I have created the table named ’employee’ which contains the employee details.
The description of the columns of ’employee’ table is listed below:
- emp_id: refers to the unique id of each employee
- emp_name: refers to the name of each employee
- email_id: refers to an email id of each employee
- city: refers to the city where the employee is situated
- Verification_status: If the verification of the background details of an employee is done, this value is stored as ‘Yes’ whereas If the verification of the background details of an employee is not done, this value is stored as ‘No’.
Suppose we want to extract specific information of employees, then we can achieve this by creating CTE.
If we want to check the verification status of employees whether it is verified or not and want to see the records of the employees whose verification is not done yet. So, we require only two columns in our CTE (temporary table) and the columns are emp_name and Verification_status containing the value as ‘No’ from the table ’employee’ .
The following code shows the example of creating CTE using the table ’employee’:
-- Creating CTE
WITH mycte AS ( SELECT emp_name , Verification_status FROM employee WHERE Verification_status = 'No'
)
SELECT * FROM mycte;
The Output is given below:
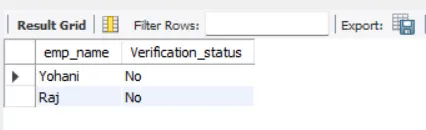
Here, ” mycte ” is the name of the CTE (temporary table). ” mycte ” contains the columns emp_name and Verification_status. It contains the details of the employees whose verification is not done yet. This is what we wanted to achieve.
We can make one or more than one CTE from the same table separated by comma.
How to Avoid Error in Common Table Expressions?
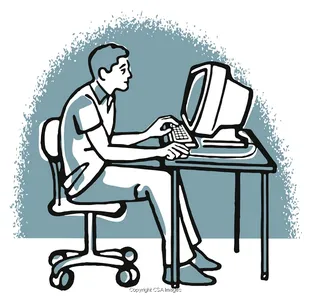
SELECT *
FROM mycte;
This SQL query is not valid. Because here in this SQL query, I cannot use “mycte” CTE.
” mycte ” CTE does not exists for this SQL query.
” mycte ” CTE exists for the only SQL query where it has been created. That is why CTE (Common Table Expression) is known as a Temporary table in SQL.
Example 2:
Now, let us understand how to use the JOIN query inside CTE. Consider the code below, which is implemented in MySQL.
-- Creating table product
CREATE TABLE product
(
p_id INT PRIMARY KEY,
p_name VARCHAR(20),
category VARCHAR(30)
); -- Creating table sales
CREATE TABLE sales
(
p_id INT PRIMARY KEY,
p_name VARCHAR(20),
gross_sales DECIMAL
); -- Inserting values into the table 'product'
INSERT INTO product VALUES (1, 'Mobile', 'Electronics');
INSERT INTO product VALUES (2, 'TV', 'Electronics');
INSERT INTO product VALUES (3, 'Car', 'Toy');
INSERT INTO product VALUES (4, 'Video game', 'Toy');
INSERT INTO product VALUES (5, 'Earphones', 'Electronics'); -- Inserting values into the table 'sales'
INSERT INTO sales VALUES (1, 'Mobile', 50000);
INSERT INTO sales VALUES (2, 'TV', 40000);
INSERT INTO sales VALUES (3, 'Car', 50000);
INSERT INTO sales VALUES (5, 'Earphones', 500000); -- Show all columns from the table 'product'
SELECT * FROM product;
-- Show all columns from the table 'product'
SELECT * FROM sales;
Output :
“product ” table:
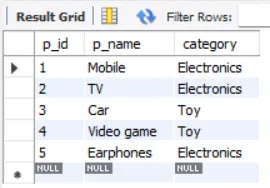
” sales ” table:
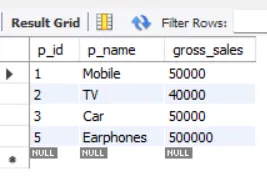
In the above example, I have created two tables ” product ” and ” sales “.
The description of the columns of the table ” product ” :
- p_id: refers to the unique ID of the product
- p_name: refers to the name of the product
- category: refers to the category to which each product belongs
The description of the columns of the table ” sales ” :
- p_id: refers to the unique ID of the product
- p_name: refers to the name of the product
- gross_sales: refers to the gross sales of each product
Then, I inserted values into the tables ” product ” and ” sales “.
-- Creating CTE
WITH TEMP_CTE AS
(
SELECT p.category AS category, COUNT(*) AS No_of_products, SUM(s.gross_sales) AS Total_gross_sales
FROM product p JOIN sales s ON p.p_id=s.p_id
GROUP BY category
ORDER BY Total_gross_sales DESC
)
SELECT * FROM TEMP_CTE;
Now, we are requested to find the gross sales in each category of the product along with the number of products in each category.
To achieve this, I created CTE named “TEMP_CTE”. In this, I have used JOIN to join the two tables “product” and “sales”. I wanted to join the tables over the records which have matches in both the tables. Hence, INNER JOIN is used. INNER JOIN is also known as JOIN. So if you write JOIN in your query instead of INNER JOIN, it will be valid only. p_id is the common column in both the tables.
To find the total gross sales and no. of products, we need to use aggregate functions sum() and count() respectively. To use the aggregate functions, GROUP BY clause has to be there. So we have grouped our result by category and find the sum of the gross sales in each category along with the product count.
Lastly, Total gross sales is ordered into descending, so that we can see the category with the highest gross sales.
Advantages of CTE
The following are the advantages of CTE:
- Common Table Expression (CTE) makes code easier to read.
- CTE can solve complex queries in an efficient manner. As an analyst who is working with SQL queries, solving complex problems is a big task and CTE helps to deal with it nicely.
- Maintaining queries and sub-queries become easier due to CTE.
- It makes the debugging of the queries easier than normal queries.
- Complex query may look simple by adding CTE inside it.
- CTE organizes the query neatly.
Conclusion
To sum up, I would like to say; Common Table Expressions is a very easy concept to implement. Some of the key takeaways are listed below:
- Using CTE makes the SQL code readable, increasing the code’s efficiency.
- CTE makes the analysis easy for analysts.
- Debugging which is one of the important parts of the queries, becomes easier due to CTE.
- To extract specific information (to be more specific, columns) from a lot of data, CTE is the most effective way to handle this situation.
If you are giving an interview for any analyst position or data scientist, the interviewer checks the knowledge of SQL. That time CTE plays a vital role in showcasing your knowledge and let the interviewer you your problem-solving skills and capabilities with greater complexity.
I hope this article about Common Table Expressions finds you insightful and helps you in your knowledgeable data journey with SQL. Hopefully, your knowledge of the amount of salt in the food has increased!
The media shown in this article is not owned by Analytics Vidhya and is used at the Author’s discretion.
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- Platoblockchain. Web3 Metaverse Intelligence. Knowledge Amplified. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2023/03/common-table-expressions-cte-in-sql/
- :is
- ][p
- $UP
- 1
- 100
- a
- About
- above
- Achieve
- advanced
- advantages
- After
- All
- always
- amount
- analysis
- analyst
- Analysts
- analytics
- Analytics Vidhya
- and
- ARE
- article
- AS
- At
- author
- avoid
- background
- BE
- because
- become
- becomes
- below
- Big
- Big Data
- by
- CAN
- cannot
- capabilities
- car
- Category
- check
- Checks
- City
- code
- Coding
- Column
- Columns
- Common
- commonly
- complex
- complexity
- concept
- concepts
- conclusion
- condition
- Consider
- contains
- corrected
- create
- created
- Creating
- data
- data scientist
- Database
- deal
- description
- details
- discretion
- each
- easier
- easily
- easy
- Effective
- effectively
- efficiency
- efficient
- efficiently
- Electronics
- Employee
- employees
- error
- Ether (ETH)
- Even
- example
- exists
- expressions
- extract
- few
- Find
- finds
- following
- follows
- food
- For
- form
- from
- functions
- game
- get
- given
- Giving
- good
- greater
- gross
- Group
- handle
- Have
- help
- helps
- here
- highest
- hope
- Hopefully
- How
- How To
- HTTPS
- i
- ID
- immediately
- implement
- implemented
- important
- in
- increasing
- information
- instead
- Interview
- Interviewer
- Introduction
- IT
- join
- journey
- Key
- knowledge
- known
- language
- like
- Listed
- Look
- Lot
- make
- MAKES
- manner
- many
- Media
- Mobile
- more
- most
- Mumbai
- MySQL
- name
- Named
- nav
- necessary
- Need
- next
- normal
- number
- occurred
- of
- on
- ONE
- order
- organizes
- original
- Other
- output
- owned
- part
- parts
- person
- plato
- Plato Data Intelligence
- PlatoData
- position
- possible
- powerful
- primary
- problem-solving
- problems
- Product
- Products
- protected
- published
- quickly
- Read
- records
- refers
- requested
- require
- required
- result
- Role
- s
- sales
- salt
- same
- Science
- Scientist
- scientists
- selected
- selecting
- Short
- should
- show
- showcasing
- shown
- Shows
- Simple
- simplify
- situation
- skills
- So
- SOLVE
- Solving
- some
- Source
- specific
- SQL
- Status
- stepping
- stored
- structured
- syntax
- table
- Takeaways
- Task
- temporary
- that
- The
- the world
- their
- Them
- There.
- Third
- time
- to
- too
- tools
- Total
- toy
- tv
- understand
- unique
- us
- use
- value
- Values
- Verification
- verified
- Video
- video game
- vital
- wanted
- Way..
- WELL
- What
- whether
- which
- WHO
- will
- with
- working
- world
- would
- write
- Your
- zephyrnet