Introduction
In Python, the ==
and is
operators are often used for comparison by programmers. Although they might seem similar at first glance, they are understood to operate differently, and their differences are considered important for anyone coding in Python to grasp. We will discuss in this article how each of these operators function and what distinguishes them from one another in detail.
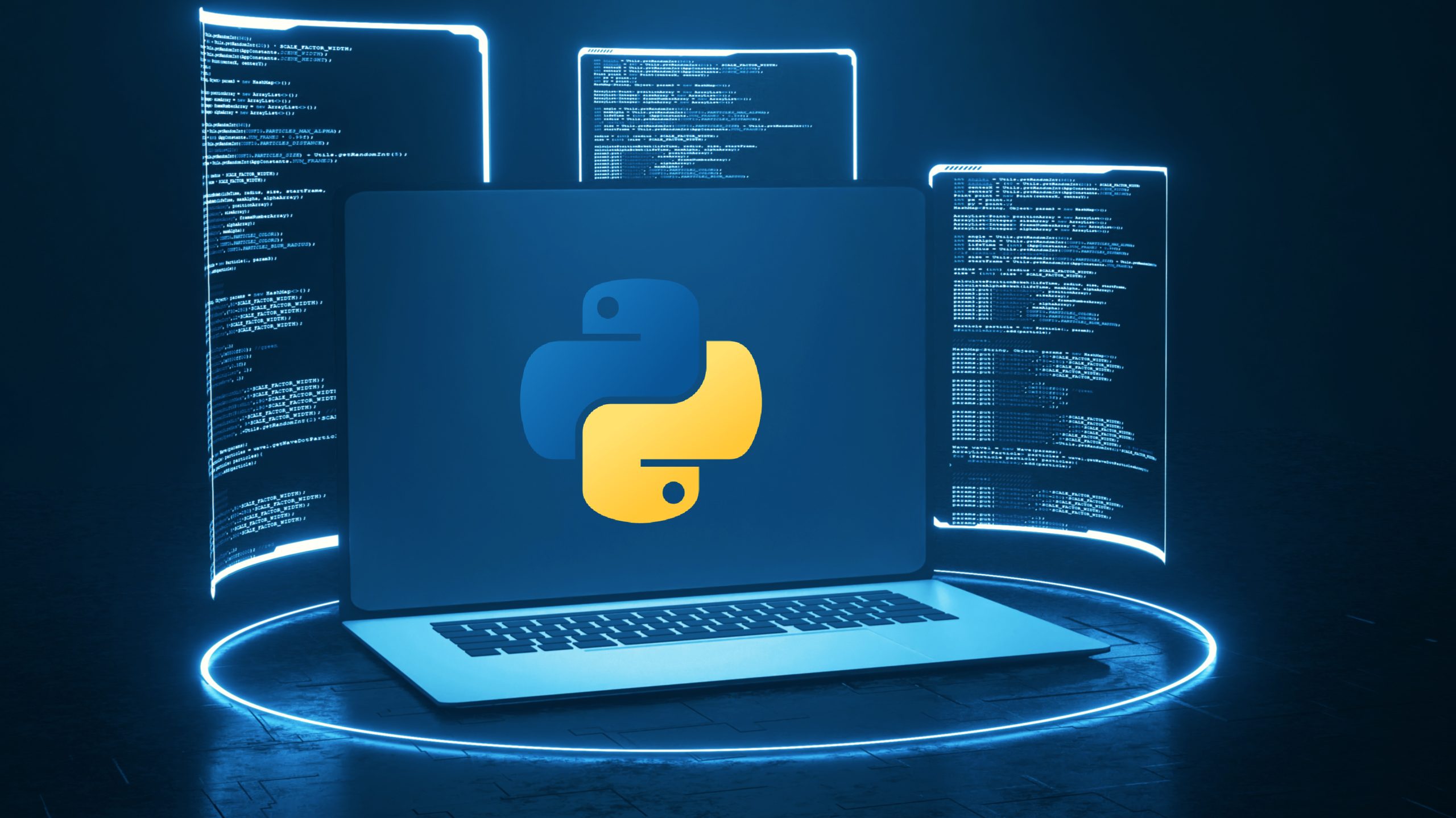
Table of Contents
Understanding the ==
Operator
The ==
operator in Python compares the values of two objects. It determines whether the values of the objects on either side of the operator are equal or not. Let us understand this with an example:
```python
x = 5
y = 5
print(x == y) # Output: True
```
Exploring the is
Operator
On the other hand, the is
operator in Python is used to check if two variables point to the same object in memory. It compares the memory addresses of the objects. For example:
```python
a = [1, 2, 3]
b = a
print(a is b) # Output: True
```
Key Differences Between == and is Operators
Here are the 3 main differences between == and is operators in Python.
1. Comparison of Values vs. Comparison of Identities
The key distinction between the == and is operators centers on their comparison focus. While the == operator is used to compare the values of the objects, The is operator however compares the memory addresses of the objects.
2. Memory Address Comparison
When using the is operator, Python checks if two variables point to the same object in memory. This can be useful when dealing with mutable objects like lists or dictionaries where you want to ensure that changes to one variable reflect in another.
3. Usage in Conditional Statements
When working with conditional statements, selecting the appropriate operator depending on your objective is crucial. For verifying whether values are equal, the == operator should be used. Conversely, the is operator should be chosen when determining whether two variables refer to the identical object.
Common Pitfalls and Misconceptions
Here are some of the most common pitfalls and misconceptions regarding the two operators in Python.
Mutable vs. Immutable Objects
One common pitfall when using the is operator is with mutable objects like lists. Since lists are mutable, even if two lists have the same values, they may not point to the same object in memory. This can lead to unexpected results when using the is operator.
Behavior with Strings and Integers
When dealing with immutable objects like strings and integers, the behavior of the is operator is more predictable. Since immutable objects cannot be changed, Python may optimize memory usage by reusing the same object for equal values.
Performance Considerations
In terms of performance, the is operator is generally faster than the == operator because it compares memory addresses directly. However, the difference in performance may not be significant for most applications unless dealing with a large number of objects.
Best Practices for Using == and is Operators
When dealing with None or Boolean values, it is recommended to use the is operator for identity comparison. Since None and boolean values are singletons in Python, using the is operator ensures consistent behavior.
Conclusion
The ==
and is
operators serve distinct purposes in Python: ==
compares the values of objects for equality, while is
checks if two variables reference the same object in memory. Understanding their differences is vital for accurate value and identity comparisons, especially with mutable and immutable objects. Correctly choosing between these operators allows for more efficient and bug-free code, particularly when handling None and Boolean values. Mastery of these concepts is crucial for robust Python programming.
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/04/difference-between-and-is-operator-in-python/
- :is
- :not
- :where
- 1
- 2%
- a
- accurate
- address
- addresses
- allows
- Although
- an
- and
- Another
- anyone
- applications
- appropriate
- ARE
- article
- At
- b
- BE
- because
- behavior
- between
- by
- CAN
- cannot
- Centers
- changed
- Changes
- check
- Checks
- choosing
- chosen
- code
- Coding
- Common
- compare
- comparison
- comparisons
- concepts
- conditional
- considered
- consistent
- conversely
- correctly
- crucial
- dealing
- Depending
- detail
- determines
- determining
- difference
- differences
- differently
- directly
- discuss
- distinct
- distinction
- distinguishes
- each
- efficient
- either
- ensure
- ensures
- equal
- equality
- especially
- Ether (ETH)
- Even
- example
- faster
- First
- Focus
- For
- from
- function
- generally
- Glance
- grasp
- hand
- Handling
- Have
- High
- How
- However
- HTTPS
- identical
- Identity
- if
- immutable
- important
- in
- integers
- IT
- jpg
- Key
- large
- lead
- let
- like
- Lists
- Main
- max-width
- May..
- Memory
- might
- misconceptions
- more
- more efficient
- most
- None
- number
- object
- objective
- objects
- of
- often
- on
- ONE
- operate
- operator
- operators
- Optimize
- or
- Other
- output
- particularly
- performance
- plato
- Plato Data Intelligence
- PlatoData
- Point
- practices
- Predictable
- Programmers
- purposes
- Python
- recommended
- refer
- reference
- reflect
- regarding
- Results
- robust
- same
- seem
- selecting
- serve
- should
- side
- significant
- similar
- since
- some
- statements
- terms
- than
- that
- The
- their
- Them
- These
- they
- this
- to
- two
- understand
- understanding
- understood
- Unexpected
- unless
- us
- Usage
- use
- used
- useful
- using
- value
- Values
- variable
- variables
- verifying
- vital
- vs
- want
- we
- What
- when
- whether
- while
- will
- with
- working
- X
- you
- Your
- zephyrnet