Introduction
Object-Oriented Programming (OOP) is a cornerstone of software development, offering a structured approach to code organization and design. Among its fundamental principles, encapsulation stands out for its ability to bundle data and the methods that operate on that data into a single cohesive unit. This article delves into the concept of encapsulation in Python, demonstrating its significance, implementation, and benefits in crafting robust, maintainable software.
Table of contents
Understanding Encapsulation
Encapsulation is akin to a protective shell that guards an object’s internal state against unintended interference and misuse. By wrapping data (attributes) and behaviors (methods) within classes and restricting access to them, encapsulation ensures a controlled interface for interaction with an object.
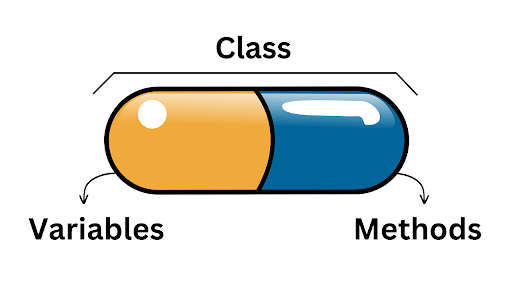
Objectives of Encapsulation
The primary goal of encapsulation is to reduce complexity and increase reusability. By hiding the internal workings of objects, developers can simplify interactions, making them more intuitive. This abstraction layer also enhances modularity, allowing for more flexible and scalable codebases.
Core Concepts of Encapsulation
Data Hiding
At the heart of encapsulation is data hiding. This concept restricts direct access to an object’s attributes, protecting its integrity by preventing external modifications unless explicitly allowed through well-defined interfaces (methods).
Access Modifiers
Unlike some languages that offer explicit access modifiers (public, protected, private), Python uses naming conventions to denote the access level of class members. The use of underscores before attribute names (_protected or __private) signals their intended access restrictions, guiding developers on their proper use.
Implementing Encapsulation in Python
Using Single and Double Underscores
Python utilizes single (_) and double (__) underscores to indicate protected and private members. Here’s how you can define them:
In this example, __balance is a private attribute, inaccessible from outside the Account class, thus encapsulating the account’s balance.
Property Decorators
Python’s property decorators (@property, @attribute.setter) provide a sophisticated mechanism for attribute access, allowing for validation and processing during assignment. Here’s an encapsulated attribute with getters and setters:
Advanced Use Case
In a banking system, encapsulation can safeguard an account’s balance, ensuring deposits and withdrawals are conducted securely, thereby maintaining the integrity of financial transactions.
Benefits of Encapsulation
- Maintaining Object Integrity: Encapsulation shields an object’s state, allowing changes through controlled operations. This protection ensures the object remains in a valid state throughout its lifecycle.
- Facilitating Code Maintenance and Scalability: By abstracting the internal details of objects, encapsulation makes code easier to manage and extend. Changes to the internal workings of a class do not affect external code, enabling smoother evolution of software systems.
Common Mistakes and Best Practices
Overusing Private Members: While privacy is a cornerstone of encapsulation, overuse can lead to rigid code structures that hinder extensibility. Use private attributes judiciously, balancing the need for protection with the flexibility for future development.
Best Practices for Encapsulation
- Use encapsulation to define clear interfaces for your classes.
- Apply property decorators to control access and validate data.
- Keep the public interface of your classes minimal to reduce coupling and enhance modularity.
Conclusion
In conclusion, encapsulation in Python is a fundamental concept that plays a crucial role in developing clean, maintainable, and robust applications. By allowing developers to bundle data and methods within a single unit and control access to that data, encapsulation enhances data integrity, reduces complexity, and improves code reusability. Using single and double underscores to denote protected and private members, alongside the powerful feature of property decorators, provides a flexible yet robust system for implementing encapsulation in Python.
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/02/encapsulation-in-python/
- :is
- :not
- 288
- a
- ability
- abstraction
- access
- affect
- against
- allowed
- Allowing
- alongside
- also
- among
- an
- and
- applications
- approach
- ARE
- article
- assignment
- attributes
- Balance
- balancing
- Banking
- banking system
- before
- behaviors
- benefits
- BEST
- Bundle
- by
- CAN
- Changes
- class
- classes
- clean
- clear
- code
- cohesive
- complexity
- concept
- concepts
- conclusion
- conducted
- control
- controlled
- Conventions
- cornerstone
- crucial
- data
- define
- delves
- demonstrating
- deposits
- Design
- details
- developers
- developing
- Development
- direct
- Direct access
- do
- double
- during
- easier
- enabling
- encapsulated
- enhance
- Enhances
- ensures
- ensuring
- evolution
- example
- explicitly
- extend
- external
- Feature
- financial
- Flexibility
- flexible
- For
- from
- fundamental
- future
- goal
- guiding
- Heart
- hiding
- High
- hinder
- How
- HTTPS
- implementation
- implementing
- improves
- in
- inaccessible
- Increase
- indicate
- integrity
- intended
- interaction
- interactions
- Interface
- interfaces
- interference
- internal
- into
- intuitive
- ITS
- Languages
- layer
- lead
- Level
- lifecycle
- Maintainable
- maintaining
- maintenance
- MAKES
- Making
- manage
- max-width
- mechanism
- Members
- methods
- minimal
- mistakes
- misuse
- Modifications
- more
- names
- naming
- Need
- object
- objects
- of
- offer
- offering
- on
- operate
- Operations
- or
- organization
- out
- outside
- plato
- Plato Data Intelligence
- PlatoData
- plays
- powerful
- practices
- preventing
- primary
- principles
- privacy
- private
- processing
- Programming
- proper
- property
- protected
- protecting
- protection
- Protective
- provide
- provides
- public
- Python
- reduce
- reduces
- remains
- restricting
- restrictions
- rigid
- robust
- Role
- safeguard
- scalable
- securely
- Shell
- signals
- significance
- simplify
- single
- smoother
- Software
- software development
- some
- sophisticated
- stands
- State
- structured
- structures
- system
- Systems
- that
- The
- their
- Them
- thereby
- this
- Through
- throughout
- Thus
- to
- Transactions
- underscores
- unit
- unless
- use
- uses
- using
- utilizes
- valid
- VALIDATE
- validation
- well-defined
- with
- Withdrawals
- within
- workings
- wrapping
- yet
- you
- Your
- zephyrnet