Introduction
Python, a versatile and powerful programming language, comes with its unique challenges, and one that developers often encounter is the AttributeError. In this comprehensive guide, we will delve into the various types of attribute errors in Python and explore the best practices for handling them. Whether you’re a novice or a seasoned Python developer, this guide is your companion to mastering the skill of managing attribute errors effectively.
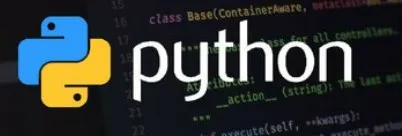
Table of contents
Types of Attribute Errors
Types of Attribute Errors: When working with Python, you may encounter diverse attribute errors, each demanding a nuanced approach. Understanding these errors is pivotal for crafting robust and error-resistant code. Let’s explore some prevalent types of attribute errors and how to tackle them.
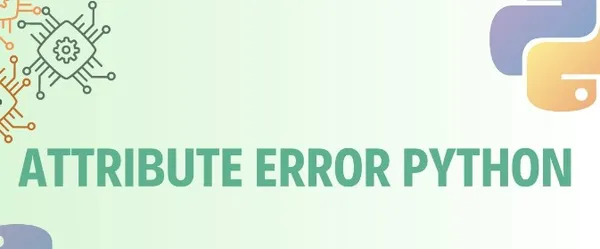
1. Accessing Nonexistent Attributes
Attempting to access an attribute that doesn’t exist for the object’s type can lead to AttributeErrors.
For instance:
marks_list = [90, 100, 95]
marks_list.append(92) # Correct usage of append() instead of add()
print(marks_list)
Output:
—————————————————————————
AttributeError Traceback (most recent call last)
<ipython-input-20-9e43b1d2d17c> in <cell line: 2>()
1 marks_list = [90,100,95]
—-> 2 marks_list.add(92)
AttributeError: ‘list’ object has no attribute ‘add’
Explanation:- Lists don’t have an add attribute to add an element; they have an append() method.
Handling Above Error by using append() method
marks_list = [90,100,95]
marks_list.add(92)
print(marks_list)
Output:
[90, 100, 95, 92]
2. Typos or Misspelled Attributes
Incorrectly spelling the attribute name is a common mistake that results in AttributeErrors.
Example:
my_str = "Hi, Himanshu."
print(my_str.low())
Output:
—————————————————————————
AttributeError Traceback (most recent call last)
<ipython-input-29-47d1e641106e> in <cell line: 2>()
1 my_str=”Hi, Himanshu.”
—-> 2 print(my_str.low())
AttributeError: ‘str’ object has no attribute ‘low’
Explanation:
The correct attribute is lower().
Handling Above Error by using lower() method
my_str="Hi, Himanshu."
print(my_str.lower())
Output:
hi, himanshu.
3. Incorrect Object Type
Expecting an object to have attributes belonging to a different type can result in AttributeErrors.
For example:
num = 42
num.upper()
Output:
—————————————————————————
AttributeError Traceback (most recent call last)
<ipython-input-30-6782a28ddc25> in <cell line: 2>()
1 num = 42
—-> 2 num.upper()
AttributeError: ‘int’ object has no attribute ‘upper’
Explanation:
The upper() method is for strings, not numbers.
Handling Above Error by using upper() method with string
num = "My Marks : 42"
num.upper()
Output:
‘MY MARKS : 42’
Also Read: Top 31 Python Projects | Beginner to Advanced (Updated 2024)
Handling Attribute Errors in Object-Oriented Programming
Object-oriented programming (OOP) in Python introduces additional nuances in handling attribute errors. Here are best practices for managing attribute errors in an OOP paradigm:
Pre-Check Existence:
Use hasattr(object, attribute_name) to verify existence before accessing.
if hasattr(object, attribute_name): value = object.attribute
Exception Handling:
Enclose attribute access in try-except blocks to catch AttributeErrors gracefully:
try: value = object.attribute
except AttributeError: # A compass for gracefully handling the error, like setting a default value or logging a warning
Safe Attribute Access:
Use getattr(object, attribute_name, default_value) for safe access and defaults:
name = getattr(person, 'name', 'Unknown')
Also Read: 7 Ways to Remove Duplicates from a List in Python
Best Practices for Handling Attribute Errors
Attribute errors can be challenging, but adopting best practices can lead to more reliable and maintainable code. Here are some guidelines:
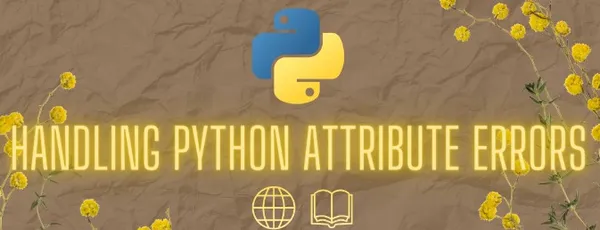
Prevention:
- Leverage type hints and IDE autocompletion for early detection of potential errors.
- Document expected attributes and methods clearly to avoid misinterpretations.
- Analyze and refactor code to minimize unnecessary attribute access attempts.
Pre-checking:
- Use hasattr(object, attribute_name) to confirm the existence of an attribute before attempting to access it. This practice eliminates the need for redundant error checks, enhancing code readability.
Exception handling:
- try-except Blocks: Safeguard attribute access by enclosing it in try-except blocks. This ensures graceful error handling. Offer clear error messages and manage the situation appropriately, such as setting a default value or logging a warning.
- Specific Exceptions: Enhance precision by catching specific AttributeError subtypes. This allows for more nuanced and targeted error handling.
Safe Access:
- Use getattr(object, attribute_name, default_value) to securely access attributes, providing a fallback value if the attribute is absent. This approach prevents dependence on potentially incorrect assumptions regarding attribute existence.
Custom Attribute Handling:
- The __getattr__() and __getattribute__() methods empower you to specify custom actions when an attribute is not located. This proves valuable for offering alternative implementations or adeptly managing unforeseen attributes.
Additional Considerations
- Dynamic Attributes: Exercise caution when dealing with dynamically generated attributes. Implement checks or safeguards to avoid accessing non-existent ones.
- Duck Typing: Prioritize flexibility, but confirm that objects adhere to the expected interface to sidestep runtime errors during method calls.
- Testing: Rigorously test your code with diverse object types and scenarios to unveil any potential attribute-related issues.
- Debugging: Employ debugging tools to trace the execution flow and identify the origin of AttributeErrors.
Conclusion
In conclusion, mastering the art of handling attribute errors is crucial for becoming a proficient Python developer. By understanding the different types of attribute errors and adopting effective strategies to handle them, you can craft more robust and error-free code. Whether you’re a novice or an experienced developer, the tips and techniques discussed in this guide will empower you to become a more confident Python programmer.
Join our free Python course and effortlessly enhance your programming prowess by mastering essential sorting techniques. Start today for a journey of skill development!
Frequently Asked Questions
A. AttributeError in Python occurs when there is an attempt to access or modify an attribute that an object does not possess or when there’s a mismatch in attribute usage.
A. Prevent the error by checking if the attribute exists using hasattr(object, attribute_name). Alternatively, employ a try-except block to gracefully handle the error and provide fallback mechanisms.
A. Common attribute errors include accessing nonexistent attributes, typos or misspelled attributes, and expecting attributes from an incorrect object type. Solutions involve pre-checking attribute existence, employing try-except blocks, and ensuring alignment with the correct object types.
A. In OOP, attribute errors can be managed by pre-checking attribute existence with hasattr, using try-except blocks for graceful error handling, and employing safe attribute access with getattr(object, attribute_name, default_value).
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/01/python-attribute-errors/
- :has
- :is
- :not
- 1
- 100
- 11
- 13
- 14
- 2024
- 31
- 7
- 8
- 90
- a
- above
- absent
- access
- accessing
- actions
- add
- Additional
- adhere
- Adopting
- advanced
- alignment
- allows
- alternative
- an
- and
- any
- approach
- appropriately
- ARE
- Art
- AS
- asked
- assumptions
- attempt
- attempting
- Attempts
- attributes
- avoid
- BE
- become
- becoming
- before
- Beginner
- belonging
- BEST
- best practices
- Block
- Blocks
- but
- by
- call
- Calls
- CAN
- Catch
- catching
- caution
- cell
- challenges
- challenging
- checking
- Checks
- clear
- clearly
- code
- comes
- Common
- companion
- Compass
- comprehensive
- conclusion
- confident
- Confirm
- correct
- craft
- crucial
- custom
- dealing
- Default
- defaults
- delve
- demanding
- dependence
- Detection
- Developer
- developers
- different
- discussed
- diverse
- do
- does
- Doesn’t
- Dont
- duplicates
- during
- dynamically
- each
- Early
- Effective
- effectively
- effortlessly
- element
- eliminates
- employing
- empower
- encounter
- enhance
- enhancing
- ensures
- ensuring
- error
- Errors
- essential
- Ether (ETH)
- example
- Except
- execution
- Exercise
- exist
- existence
- exists
- expected
- expecting
- experienced
- explore
- Fix
- Flexibility
- flow
- For
- from
- generated
- Graceful
- guide
- guidelines
- handle
- handled
- Handling
- Have
- here
- hi
- hints
- How
- How To
- HTTPS
- i
- identify
- if
- implement
- implementations
- in
- include
- instance
- instead
- Interface
- into
- Introduces
- involve
- issues
- IT
- ITS
- journey
- language
- Last
- lead
- like
- Line
- List
- Lists
- located
- logging
- Maintainable
- manage
- managed
- managing
- Mastering
- May..
- mechanisms
- messages
- method
- methods
- minimize
- mistake
- modify
- more
- most
- my
- name
- Need
- no
- nonexistent
- novice
- nuances
- numbers
- object
- objects
- of
- offer
- offering
- often
- on
- ONE
- ones
- or
- Origin
- our
- paradigm
- person
- pivotal
- plato
- Plato Data Intelligence
- PlatoData
- possess
- potential
- potentially
- powerful
- practice
- practices
- Precision
- prevalent
- prevent
- prevents
- Prioritize
- Programmer
- Programming
- projects
- proves
- provide
- providing
- prowess
- Python
- Python developer
- Read
- recent
- redundant
- Refactor
- regarding
- reliable
- remove
- resolved
- result
- Results
- robust
- runtime
- safe
- safeguards
- scenarios
- seasoned
- securely
- setting
- situation
- skill
- Solutions
- some
- specific
- spelling
- start
- strategies
- such
- tackle
- targeted
- techniques
- test
- that
- The
- Them
- There.
- These
- they
- this
- tips
- to
- today
- tools
- trace
- type
- types
- understanding
- unforeseen
- unique
- unknown
- unnecessary
- unveil
- updated
- Usage
- using
- Valuable
- value
- various
- verify
- versatile
- warning
- ways
- we
- webp
- What
- What is
- when
- whether
- will
- with
- working
- you
- Your
- zephyrnet