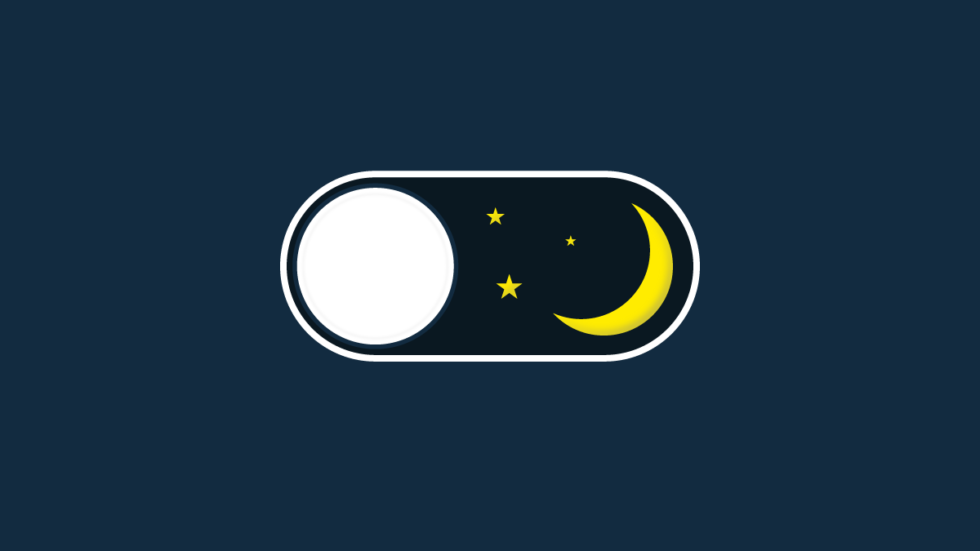
NOTE: This tutorial is relevant to developers using a CSS-in-JS styling approach, e.g. Emotion.js, Material UI, etc.
Step 1: Use Recoil atom for the currently selected theme’s name
import { PaletteMode } from "@mui/material";
import { atom } from "recoil"; export const ThemeName = atom<PaletteMode>({ key: "ThemeName", effects: []
});
Step 2: Use Recoil selector for the currently selected theme object
import { createTheme } from "@mui/material";
import { selector } from "recoil"; export const Theme = selector({ key: "Theme", dangerouslyAllowMutability: true, get(ctx) { const name = ctx.get(ThemeName); return createTheme(); },
});
Step 3: Add useTheme()
, useToggleTheme()
React hooks
import { useRecoilValue, useRecoilCallback } from "recoil"; export function useTheme() { return useRecoilValue(Theme);
} export function useToggleTheme() { return useRecoilCallback( (ctx) => () => { ctx.set(ThemeName, (prev) => (prev === "dark" ? "light" : "dark")); }, [] );
}
Step 4: Save/restore the currently selected theme name to/from localStorage
export const ThemeName = atom<PaletteMode>({ key: "ThemeName", effects: [ (ctx) => { const storageKey = "theme"; if (ctx.trigger === "get") { const name: PaletteMode = localStorage?.getItem(storageKey) === "dark" ? "dark" : localStorage?.getItem(storageKey) === "light" ? "light" : matchMedia?.("(prefers-color-scheme: dark)").matches ? "dark" : "light"; ctx.setSelf(name); } ctx.onSet((value) => { localStorage?.setItem(storageKey, value); }); }, ],
});
Step 5: Add ThemeProvider
to the top-level React component
import { ThemeProvider } from "@mui/material";
import { useTheme } from "../theme/index.js"; function App(): JSX.Element { const theme = useTheme(); return ( <ThemeProvider theme={theme}> {/* ... */} </Theme> );
}
See app/theme/index.ts
in React Starter Kit
Resources
- SEO Powered Content & PR Distribution. Get Amplified Today.
- Platoblockchain. Web3 Metaverse Intelligence. Knowledge Amplified. Access Here.
- Source: https://www.codementor.io/koistya/how-to-implement-ui-theme-switching-dark-mode-with-react-and-recoil-1vvjynmi1e
More from Codementor React Fact
Showing a modal dialog with useImperativeHandle() React hook
Source Node: 1778496
Time Stamp: Sep 17, 2022
Simple Introduction to Unit Testing in React.js in Plain English | Medium
Source Node: 1991911
Time Stamp: Mar 4, 2023
Effective Performance Optimization Techniques for Frontend Development | Codementor
Source Node: 2125812
Time Stamp: Jun 8, 2023
Computer science student unblocking their project with Chat GPT
Source Node: 2001450
Time Stamp: Mar 9, 2023
Intern’s Guide Chat GPT Full Stack: Nest, React, Typescript
Source Node: 1996513
Time Stamp: Mar 6, 2023
Man, Machine, and the Art of Algorithmic Mastery: A GPT4 Perspective
Source Node: 2010924
Time Stamp: Mar 14, 2023