Introduction
Python dictionaries are a powerful data structure that allows you to store key-value pairs. However, there are times when you need to remove a key from a dictionary. In this blog, we will explore 4 ways to remove a key from a dictionary in Python.
Table of contents
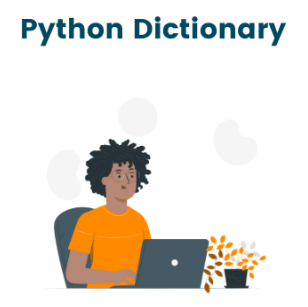
Using the pop() Method
The pop() method is a built-in function in Python that removes a key from a dictionary and returns its value. Here’s an example of how to use the pop() method to remove a key from a dictionary:
Example:
# Create a dictionary my_dict = {'a': 1, 'b': 2, 'c': 3} # Remove the key 'a' from the dictionary value = my_dict.pop('a') # Print the updated dictionary print(my_dict)
Output:
{‘b’: 2, ‘c’: 3}
Using the del Keyword
Another way to remove a key from a dictionary is by using the del keyword.
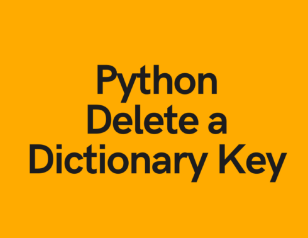
Here’s an example of how to use the del keyword to remove a key from a dictionary:
Example:
# Create a dictionary my_dict = {'a': 1, 'b': 2, 'c': 3} # Remove the key 'a' from the dictionary del my_dict['a'] # Print the updated dictionary print(my_dict)
Output:
{‘b’: 2, ‘c’: 3}
Using the popitem() Method
The popitem() method is another built-in function in Python that removes the last key-value pair from a dictionary. Here’s an example of how to use the popitem() method to remove a key from a dictionary:
Example:
# Create a dictionary my_dict = {'a': 1, 'b': 2, 'c': 3} # Remove the last key-value pair from the dictionary my_dict.popitem() # Print the updated dictionary print(my_dict)
Output:
{‘a’: 1, ‘b’: 2}
Using the Dictionary Comprehension
Another way to remove a key from a dictionary is by using dictionary comprehension. Here’s an example of how to use dictionary comprehension to remove a key from a dictionary:
Example:
# Create a dictionary my_dict = {'a': 1, 'b': 2, 'c': 3} # Remove the key 'a' from the dictionary new_dict = {key: value for key, value in my_dict.items() if key != 'a'} # Print the updated dictionary print(new_dict)
Output:
{‘b’: 2, ‘c’: 3}
Conclusion
In this blog, we explored 4 ways to remove a key from a Python dictionary. Whether you prefer using built-in methods like pop() and del, or popitem(), there are plenty of options to choose from when it comes to removing a key from a dictionary. By understanding these different methods, you can become a more effective Python programmer and handle dictionary manipulation with ease.
You can also enroll in our Free Courses Today!
You can also read more articles related to Python Lists here:
Frequently Asked Questions
Q1. How can I remove a key from a dictionary in Python?
A. You can remove a key from a dictionary using the pop()
method or the del
statement.
A. Yes, use the pop()
method with a default value or the dict.get()
method to avoid errors when removing a key that might not exist.
A. Yes, you can use the popitem()
method to remove and return the last key-value pair from the dictionary.
A. You can use the clear()
method to remove all key-value pairs from a dictionary, leaving it empty.
A. Use the pop()
method with the key you want to remove. It returns the value associated with the key while removing it from the dictionary.
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/02/ways-to-remove-a-key-from-a-dictionary-in-python/
- :is
- :not
- 1
- 13
- 2%
- 4
- 48
- 5
- 7
- 8
- 9
- a
- All
- allows
- also
- an
- and
- Another
- ARE
- articles
- asked
- associated
- At
- avoid
- b
- become
- Blog
- built-in
- by
- CAN
- Choose
- clear
- comes
- create
- data
- Default
- del
- different
- do
- ease
- Effective
- empty
- enroll
- error
- Errors
- example
- exist
- explore
- Explored
- For
- from
- function
- get
- handle
- here
- High
- How
- How To
- However
- HTTPS
- i
- if
- in
- IT
- ITS
- Key
- keys
- keyword
- Knowing
- Last
- leaving
- like
- Lists
- Manipulation
- max-width
- method
- methods
- might
- more
- Need
- of
- Options
- or
- pair
- pairs
- plato
- Plato Data Intelligence
- PlatoData
- Plenty
- powerful
- prefer
- present
- Programmer
- Python
- raising
- Read
- related
- remove
- removes
- removing
- return
- returns
- safely
- same
- Statement
- store
- structure
- SVG
- that
- The
- There.
- These
- this
- time
- times
- to
- understanding
- updated
- use
- using
- value
- want
- Way..
- ways
- we
- What
- when
- whether
- while
- will
- with
- without
- yes
- you
- zephyrnet