Introduction
Python is renowned for its easy-to-understand syntax and powerful data structures, with dictionaries being among the most versatile and widely used. However, developers often encounter a common exception when working with dictionaries: KeyError. Understanding why KeyError exceptions occur and knowing how to handle them effectively is crucial for writing robust Python code.
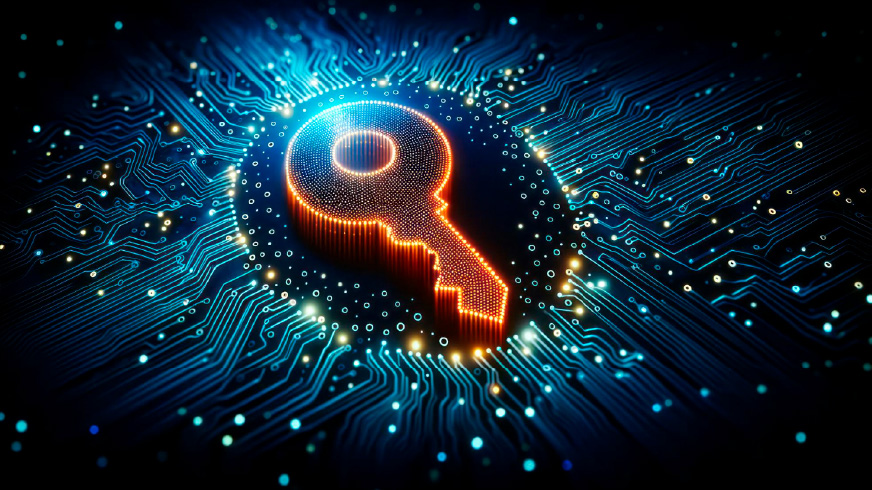
Table of contents
Understanding KeyError in Depth
A KeyError in Python is raised when trying to access a dictionary key that does not exist. This exception is a part of Python’s built-in exceptions hierarchy, indicating errors related to key operations in mapping types like dictionaries.
Common Scenarios Leading to KeyError
KeyError exceptions can arise in various situations, such as:
- Directly accessing a key that doesn’t exist in the dictionary.
- Iterating over a dictionary and attempting to access keys that might have been removed or modified during the iteration.
- Misapplying dictionary methods, for instance, misunderstanding the behavior of dict[key] versus dict.get(key).
Preventative Measures to Avoid KeyError
Prevention is better than cure, especially in programming. Here are techniques to preemptively avoid KeyError:
Checking if a Key Exists
Using the in keyword or the .get() method provides safer ways to access dictionary values:
- The in-keyword checks for the existence of a key before access.
- If the key is not found, the .get() method returns a default value (None by default), avoiding the exception.
Handling KeyError Exceptions with Try-Except
When preventive measures are not applicable, handling exceptions using try-except blocks is a practical approach:
<script src="https://gist.github.com/harshitahluwalia7895/1dad66df33769b8eed993c71c21cbe99.js"></script>
This method ensures the program can continue running even if a KeyError is encountered.
Also read: A Complete Python Tutorial to Learn Data Science from Scratch
Using DefaultDict to Avoid KeyError
The collections.defaultdict class provides a default value for the key that does not exist:
from collections import defaultdict
<script src="https://gist.github.com/harshitahluwalia7895/78c362990e9c3f87b989aafb4d853666.js"></script>
Advanced Strategies for KeyError Handling
Custom Exception Handling
Creating custom exceptions can provide more context about the error:
<script src="https://gist.github.com/harshitahluwalia7895/ba3c19721e2556a8a60078fa7ee1d4f8.js"></script>
Logging KeyError Exceptions
Logging exceptions rather than printing them can help in debugging and maintaining the code:
<script src="https://gist.github.com/harshitahluwalia7895/528d244aa81661fcdc4db5ac572afd30.js"></script>
Practical Tips for Working with Dictionaries Safely
- Use .get() and .setdefault() methods for safer key access and setting defaults.
- Prefer collections.defaultdict for dictionaries expected to have missing keys.
- Employ try-except blocks wisely to handle exceptions without cluttering the code.
Conclusion
Dealing with Python KeyError exceptions is a fundamental aspect of working with dictionaries in Python. By understanding why these errors occur and employing strategies to handle or avoid them, developers can ensure that their Python applications are more robust and error-resistant. Adopting best error-handling practices improves code quality and enhances the overall reliability and maintainability of Python projects.
If you are looking for a Python course online, then explore Learn Python for Data Science
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/02/python-keyerror-exceptions-and-how-to-handle-them/
- :is
- :not
- a
- About
- access
- accessing
- Adopting
- among
- and
- applicable
- applications
- approach
- ARE
- arise
- AS
- aspect
- attempting
- avoid
- avoiding
- been
- before
- behavior
- being
- BEST
- Better
- Blocks
- built-in
- by
- CAN
- Checks
- class
- cluttering
- code
- collections
- Common
- complete
- context
- continue
- course
- crucial
- cure
- custom
- data
- data science
- Default
- defaults
- developers
- DICT
- does
- Doesn’t
- during
- effectively
- employing
- encounter
- Enhances
- ensure
- ensures
- error
- Errors
- especially
- Even
- exception
- exist
- existence
- expected
- explore
- For
- found
- from
- fundamental
- GitHub
- handle
- Handling
- Have
- help
- here
- hierarchy
- High
- How
- How To
- However
- HTTPS
- if
- import
- improves
- in
- indicating
- instance
- iteration
- ITS
- jpg
- Key
- keys
- keyword
- Knowing
- leading
- LEARN
- like
- looking
- maintainability
- maintaining
- mapping
- max-width
- measures
- method
- methods
- might
- missing
- misunderstanding
- modified
- more
- most
- None
- occur
- of
- often
- online
- Operations
- or
- over
- overall
- part
- plato
- Plato Data Intelligence
- PlatoData
- powerful
- Practical
- practices
- printing
- Program
- Programming
- projects
- provide
- provides
- Python
- quality
- raised
- rather
- Read
- related
- reliability
- Removed
- Renowned
- returns
- robust
- running
- safer
- scenarios
- Science
- script
- setting
- situations
- strategies
- structures
- such
- syntax
- techniques
- than
- that
- The
- their
- Them
- then
- These
- this
- tips
- to
- trying
- tutorial
- types
- understanding
- used
- using
- value
- Values
- various
- versatile
- Versus
- ways
- when
- why
- widely
- wisely
- with
- without
- working
- writing
- you
- zephyrnet